Version aq: mouse wheel support
Lua Carousel » Devlog
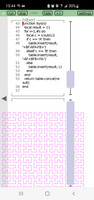
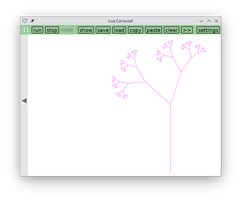
I've been focusing so much on the mobile/touchscreen experience that I forgot to wire up the mouse wheel. This omission is now fixed, along with a minor bugfix and a few more abbreviations (example 7). Thanks to LÖVE Forum user milon for reminding me of this.
This might also be a good time to share a couple of programs I've been building using Lua Carousel over the past week. As you copy them and paste them in, remember to load the abbreviations before running them.
A hilbert curve
x,y = 0,700 dirx,diry = 1,0 n = 10 g.setLineWidth(3) color(1,0,1, 0.1) function draw_lsys(s) for i=1,#s do local c = s:sub(i,i) if c == 'F' then forward() elseif c == '+' then left() elseif c == '-' then right() end end end function forward() local x2 = x+dirx*n local y2 = y+diry*n line(x,y, x2,y2) x,y = x2,y2 end function left() if dirx == 0 then dirx = diry diry = 0 else diry = -dirx dirx = 0 end end function right() if dirx == 0 then dirx = -diry diry = 0 else diry = dirx dirx = 0 end end function lsys(s) local result = {} for i=1,#s do local c = s:sub(i,i) if c == 'A' then table.insert(result, '+BF-AFA-FB+') elseif c == 'B' then table.insert(result, '-AF+BFB+FA-') else table.insert(result, c) end end return table.concat(result) end s = 'A' for _ = 1,5 do s = lsys(s) end draw_lsys(s)
A tree fractal
Iters = 7 Zoom = 10 X0 = Safe_width*3/4 Y0 = Safe_height-10 N = Safe_height/2/Iters/Zoom Left = -0.3 Right = 0.7 function car.draw() x,y = X0,Y0 Angle = -pi/2 Stack = {} color(1,0,1, 0.4) s = '0' for _ = 1,Iters do s = lsys(s) end --print(s) draw_lsys(s) end function draw_lsys(s) for i=1,#s do local c = s:sub(i,i) if c == '0' or c == '1' then forward() elseif c == '[' then push() elseif c == ']' then pop() end end end function forward() local x2 = x + N*cos(Angle) local y2 = y + N*sin(Angle) line(x,y, x2, y2) --print('|', x,y, x2,y2) x, y = x2, y2 end function push() table.insert(Stack, {x, y, Angle}) Angle = Angle+Left --print('[', x,y, Angle) end function pop() x,y, Angle = unpack(Stack[#Stack]) table.remove(Stack) Angle = Angle+Right --print(']', x,y, Angle) end function lsys(s) local result = {} for i=1,#s do local c = s:sub(i,i) if c == '1' then table.insert(result, '11') elseif c == '0' then table.insert(result, '1[0]0') else table.insert(result, c) end end return table.concat(result) end function car.mouse_press(x,y, b) --X0,Y0 = x,y Left = -x/Safe_width Right = y/Safe_height end function car.mouse_wheel_move(dx,dy) Iters = Iters+dy Iters = math.max(Iters, 1) Iters = math.min(Iters, 12) N = Safe_height/2/Iters/Zoom end
To connect up with the start of this post, try hiding the editors and then moving the mouse wheel on the tree fractal.
Files
carousel-aq.love 108 kB
Dec 02, 2023
carousel-aq-safe.love 108 kB
Dec 02, 2023
Get Lua Carousel
Lua Carousel
Write programs on desktop and mobile
Status | In development |
Category | Tool |
Author | Kartik Agaram |
Tags | LÖVE |
More posts
- Visualizing the digits of π4 days ago
- A little recursion problem23 days ago
- Drawing histograms35 days ago
- The simplest possible dither39 days ago
- All the 1-D cellular automata46 days ago
- A Sokoban client based on Lua Carousel58 days ago
- Lots of charts74 days ago
- A little programming game77 days ago
- New version after 51 days81 days ago
- Pong Wars99 days ago
Leave a comment
Log in with itch.io to leave a comment.