Playing with LÖVE's physics engine for the first time
Lua Carousel » Devlog
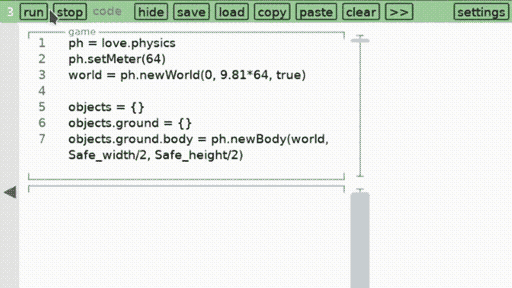
I thought I'd make sure today that Lua Carousel correctly handles LÖVE's physics libraries. No issues! And then my son suggested I could turn the example in the LÖVE tutorial into a very simple game. Not for a touchscreen, unfortunately. Here's the code:
ph = love.physics ph.setMeter(64) world = ph.newWorld(0, 9.81*64, true) objects = {} objects.ground = {} objects.ground.body = ph.newBody(world, Safe_width/2, Safe_height/2) objects.ground.shape = ph.newRectangleShape(Safe_width-200, 50) objects.ground.fixture = ph.newFixture(objects.ground.body, objects.ground.shape) objects.ball = {} objects.ball.body = ph.newBody(world, Safe_width/2,0, "dynamic") objects.ball.shape = ph.newCircleShape(20) objects.ball.fixture = ph.newFixture(objects.ball.body, objects.ball.shape, --[[density]] 1) objects.ball.fixture:setRestitution(0.9) -- bounce objects.block1 = {} objects.block1.body = ph.newBody(world, Safe_width*3/4, Safe_height/2-100, 'dynamic') objects.block1.shape = love.physics.newRectangleShape(0, 0, 50, 100) objects.block1.fixture = love.physics.newFixture(objects.block1.body, objects.block1.shape, 1) objects.block2 = {} objects.block2.body = ph.newBody(world, Safe_width*3/4, Safe_height/2-200, 'dynamic') objects.block2.shape = ph.newRectangleShape(0, 0, 100, 50) objects.block2.fixture = ph.newFixture(objects.block2.body, objects.block2.shape, 1) function car.update(dt) world:update(dt) if objects.block1.body:getY() > Safe_height/2 and objects.block2.body:getY() > Safe_height/2 then Result = 'You win!' elseif objects.ball.body:getY() > Safe_height/2 then Result = 'You lost!' end end Result = '' function car.draw() if Result == '' then color(0.4, 0.4, 0.4) g.print('a: push left', 50,30) g.print('l: push right', 50,60) g.print('u: try again', 50,90) elseif Result:find('lost') then color(1,0,0) g.print(Result, 50,50) elseif Result:find('win') then color(0,0,1) g.print(Result, 50,50) end color(0.28, 0.63, 0.05) poly('fill', objects.ground.body:getWorldPoints(objects.ground.shape:getPoints())) color(0.76, 0.18, 0.05) circle('fill', objects.ball.body:getX(), objects.ball.body:getY(), objects.ball.shape:getRadius()) color(0.20, 0.20, 0.20) poly('fill', objects.block1.body:getWorldPoints(objects.block1.shape:getPoints())) poly('fill', objects.block2.body:getWorldPoints(objects.block2.shape:getPoints())) end function car.keypressed(key) if key == 'l' then objects.ball.body:applyForce(400, 0) elseif key == 'a' then objects.ball.body:applyForce(-400, 0) elseif key == 'u' then objects.ball.body:setPosition(Safe_width/2, 0) objects.ball.body:setLinearVelocity(0, 0) Result = '' end end
As before, you'll need to either run the abbreviations screen in the examples or paste in these up top:
g = love.graphics circle, poly = g.circle, g.polygon color = g.setColor
Get Lua Carousel
Lua Carousel
Write programs on desktop and mobile
Status | In development |
Category | Tool |
Author | Kartik Agaram |
Tags | LÖVE |
More posts
- New version after 9 days6 days ago
- New version after 3 days37 days ago
- New version after 40 days40 days ago
- Turn your phone or tablet into a chess clock54 days ago
- Guest program: bouncing balls70 days ago
- New version after 3 months, and turtle graphics81 days ago
- Interactively zooming in to the Mandelbrot set on a touchscreenSep 16, 2024
- A little timer appSep 15, 2024
- New version after 4 monthsJun 28, 2024
- Visualizing the digits of πMay 04, 2024
Leave a comment
Log in with itch.io to leave a comment.