Pushing objects around in a dozen lines of code
Lua Carousel » Devlog
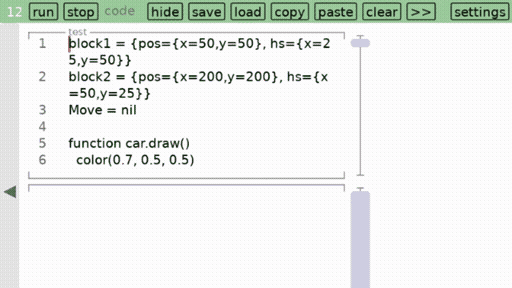
I've been playing around with detecting and responding to collisions, just with simple rectangles. Here's today's example, with lots of help from the LÖVE Discord that pointed me to the batteries library in particular:
block1 = {pos={x=50,y=50}, hs={x=25,y=50}} block2 = {pos={x=200,y=200}, hs={x=50,y=25}} Move = nil function car.draw() color(0.7, 0.5, 0.5) local r = block1 rect('fill', r.pos.x-r.hs.x,r.pos.y-r.hs.y, r.hs.x*2,r.hs.y*2) color(0.5, 0.5, 0.5) r = block2 rect('fill', r.pos.x-r.hs.x,r.pos.y-r.hs.y, r.hs.x*2,r.hs.y*2) end function car.mousepressed(x,y, button) if x < block1.pos.x - block1.hs.x then return end if x > block1.pos.x + block1.hs.x then return end if y < block1.pos.y - block1.hs.y then return end if y > block1.pos.y + block1.hs.y then return end Move = {target=block1, dx=x-block1.pos.x, dy=y-block1.pos.y} end function car.mousereleased(x,y, done) Move = nil end function car.update(dt) if Move == nil or Move.target ~= block1 then return end local x,y = love.mouse.getPosition() block1.pos.x = x-Move.dx block1.pos.y = y-Move.dy local msv = collide(block1.pos, block1.hs, block2.pos, block2.hs) if msv then block2.pos.x = block2.pos.x - msv.x block2.pos.y = block2.pos.y - msv.y end end -- returns the _minimum separation vector_ if there's a collision function collide(apos, ahs, bpos, bhs) local delta = {x=apos.x-bpos.x, y=apos.y-bpos.y} local abs_delta = {x=abs(apos.x-bpos.x), y=abs(apos.y-bpos.y)} local size = {x=ahs.x+bhs.x, y=ahs.y+bhs.y} local abs_amount = {x=size.x-abs_delta.x, y=size.y-abs_delta.y} if abs_amount.x > 0 and abs_amount.y > 0 then if abs_amount.x <= abs_amount.y then return {x=abs_amount.x*sign(delta.x), y=0} else return {x=0, y=abs_amount.y*sign(delta.y)} end end end function sign(v) if v < 0 then return -1 end if v > 0 then return 1 end return 0 end
If you try pasting this program into Lua Carousel, remember to first run the abbreviations on one of the example screens. Or if you've deleted that screen, here are the abbreviations this program uses:
g = love.graphics rect = g.rectangle color = g.setColor abs = math.abs
Get Lua Carousel
Lua Carousel
Write programs on desktop and mobile
Status | In development |
Category | Tool |
Author | Kartik Agaram |
Tags | LÖVE |
More posts
- Lua Carousel: program on the device you have, with docs at your fingertips19 days ago
- Pong Wars, MMO editionFeb 16, 2025
- New version after 41 days, and stop-motion animationFeb 15, 2025
- Drawing with a pen on a pendulumJan 11, 2025
- New version after 16 daysJan 04, 2025
- New version after 9 daysDec 19, 2024
- New version after 3 daysNov 17, 2024
- New version after 40 daysNov 14, 2024
- Turn your phone or tablet into a chess clockNov 01, 2024
- Guest program: bouncing ballsOct 15, 2024
Leave a comment
Log in with itch.io to leave a comment.