The simplest possible dither
Lua Carousel » Devlog
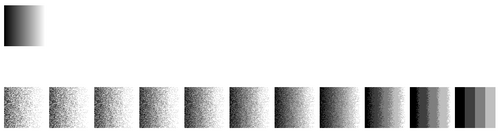
I saw this exchange yesterday:
"Is there a trick for doing dithering that is so simple you can tell it to me in 500 chars and I will instantly memorize it and then never have to look it up again?"
"Add noise before quantization."
And of course I had to play with this idea. Here's a program that creates a simple greyscale image and quantizes it to 4 levels of greyscale -- after adding varying levels of noise.
N = 100 -- image size img = {} for y=1,N do local row = {} for x=1,N do table.insert(row, x/N) end table.insert(img, row) end function add_noise_then_quantize(img, f, q) local result = {} for y=1,#img do local row = {} for x=1,#img[y] do local c = img[y][x] + love.math.noise(x,y)*f -- add noise table.insert(row, floor(c*q)/q) -- quantize end table.insert(result, row) end return result end dithered_img = {} for i=0,10 do dithered_img[i] = add_noise_then_quantize(img, i/10, 4) end function car.draw() draw_image(img, 700, 100) for i=0,10 do draw_image(dithered_img[i], 700+(10-i)*110, 300) end end function draw_image(img, x0,y0) for y=1,#img do for x=1,#img[y] do local c = img[y][x] color(c,c,c) pt(x0+x, y0+y) end end end
Get Lua Carousel
Lua Carousel
Write programs on desktop and mobile
Status | In development |
Category | Tool |
Author | Kartik Agaram |
Tags | LÖVE |
More posts
- Lua Carousel: program on the device you have, with docs at your fingertips40 days ago
- Pong Wars, MMO editionFeb 16, 2025
- New version after 41 days, and stop-motion animationFeb 15, 2025
- Drawing with a pen on a pendulumJan 11, 2025
- New version after 16 daysJan 04, 2025
- New version after 9 daysDec 19, 2024
- New version after 3 daysNov 17, 2024
- New version after 40 daysNov 14, 2024
- Turn your phone or tablet into a chess clockNov 01, 2024
- Guest program: bouncing ballsOct 15, 2024
Leave a comment
Log in with itch.io to leave a comment.